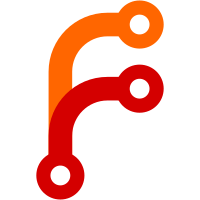
* Persists Redux store when reloading app for login * Disable confirmation box when leaving page for login * Removes extra console.warn * Sets serveSecure: true for new projects if served over HTTPS * Clears persisted state on IDEView load Because when a sketch is created on HTTPS and then the user logs in the page won't be reloaded * Appends ?source=<protocol> to URL to track return protocol
27 lines
601 B
JavaScript
27 lines
601 B
JavaScript
/*
|
|
Saves and loads a snapshot of the Redux store
|
|
state to session storage
|
|
*/
|
|
const key = 'p5js-editor';
|
|
const storage = sessionStorage;
|
|
|
|
export const saveState = (state) => {
|
|
try {
|
|
storage.setItem(key, JSON.stringify(state));
|
|
} catch (error) {
|
|
console.warn('Unable to persist state to storage:', error);
|
|
}
|
|
};
|
|
|
|
export const loadState = () => {
|
|
try {
|
|
return JSON.parse(storage.getItem(key));
|
|
} catch (error) {
|
|
console.warn('Failed to retrieve initialize state from storage:', error);
|
|
return null;
|
|
}
|
|
};
|
|
|
|
export const clearState = () => {
|
|
storage.removeItem(key);
|
|
};
|