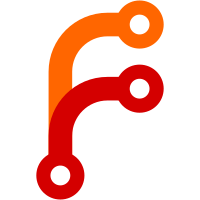
* Higher-order component to force some routes to HTTPS * Force all user-management routes to HTTPS * Redirect to sourceProtocol as route unmounts. By default, no redirection occurs if sourceProtocol is not explicitly defined. * Sets serveSecure flag on new projects and usea after forcing protocol The flag is set to `false` on all projects and as the UI has no way to change this, it always redirects to HTTP after a signup/login action. * Move HoC to be with other top-level components * Server should respond to account page request * Serves AccountView over HTTPS * Turns HTTPS redirection off in development by default Will log to the browser console any redirection that would have happened. Added a line in the README about how to enable this for testing in development.
44 lines
1.2 KiB
JavaScript
44 lines
1.2 KiB
JavaScript
import React, { PropTypes } from 'react';
|
|
|
|
/**
|
|
* A Higher Order Component that forces the protocol to change on mount
|
|
*
|
|
* targetProtocol: the protocol to redirect to on mount
|
|
* sourceProtocol: the protocol to redirect back to on unmount
|
|
* disable: if true, the redirection will not happen but what should
|
|
* have happened will be logged to the console
|
|
*/
|
|
const forceProtocol = ({ targetProtocol = 'https:', sourceProtocol, disable = false }) => WrappedComponent => (
|
|
class ForceProtocol extends React.Component {
|
|
static propTypes = {}
|
|
|
|
componentDidMount() {
|
|
this.redirectToProtocol(targetProtocol);
|
|
}
|
|
|
|
componentWillUnmount() {
|
|
if (sourceProtocol != null) {
|
|
this.redirectToProtocol(sourceProtocol);
|
|
}
|
|
}
|
|
|
|
redirectToProtocol(protocol) {
|
|
const currentProtocol = window.location.protocol;
|
|
|
|
if (protocol !== currentProtocol) {
|
|
if (disable === true) {
|
|
console.info(`forceProtocol: would have redirected from "${currentProtocol}" to "${protocol}"`);
|
|
} else {
|
|
window.location = window.location.href.replace(currentProtocol, protocol);
|
|
}
|
|
}
|
|
}
|
|
|
|
render() {
|
|
return <WrappedComponent {...this.props} />;
|
|
}
|
|
}
|
|
);
|
|
|
|
|
|
export default forceProtocol;
|